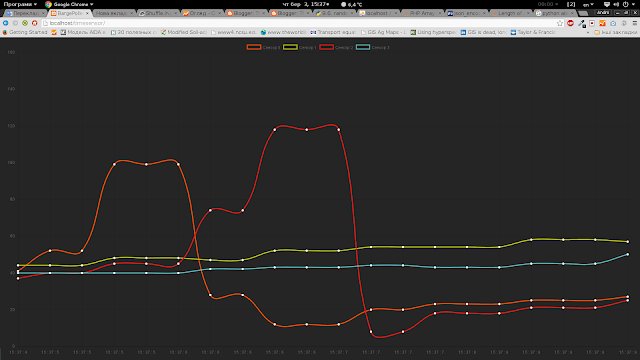
Sometimes we need to stream our yielded data into Internet, and display them, for instance, as a chart.
So, let`s go.
For instance we need to stream data from multiple sensors or devices directly to server and display them with minimal delay.
This solution could be used for smart-house systems, air quality devices, and other,...